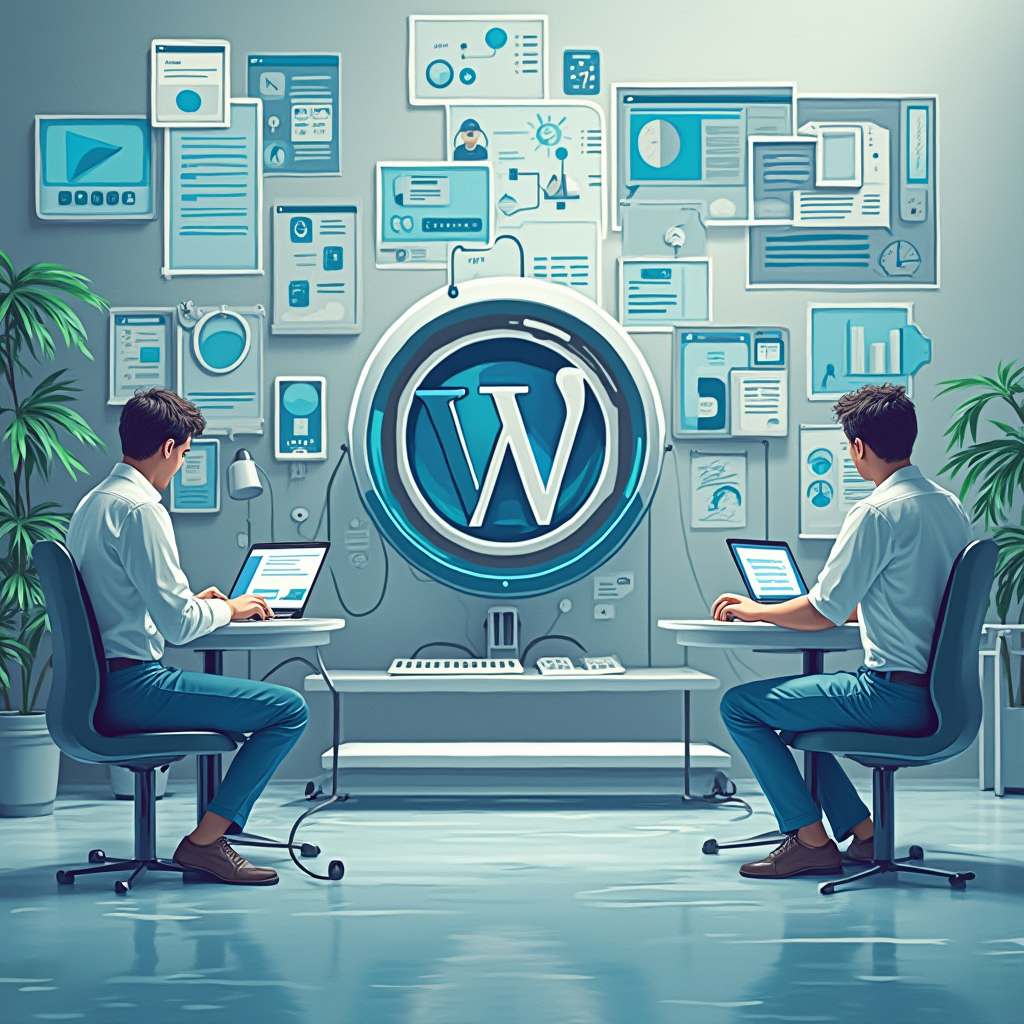
Integrating Firebase with WordPress can add powerful features such as real-time database updates, authentication, analytics, and more. Firebase’s robust backend services combined with WordPress’s flexibility make this integration a game-changer for modern web development. This guide provides a step-by-step approach to achieve Firebase and WordPress integration effectively.
Step 1: Set Up a Firebase Project
- Go to the Firebase Console.
- Click on Add Project and provide a project name.
- Follow the prompts to configure and create your Firebase project.
- Once created, you’ll be redirected to the Firebase dashboard.
Step 2: Configure Firebase Services
Firebase offers several services that can integrate with WordPress:
- Authentication: Allow users to sign up or log in via email, Google, or other providers.
- Firestore Database: Real-time storage and synchronization of data.
- Hosting: Deploy static assets.
- Cloud Functions: Run server-side logic.
- Analytics: Track user activity.
Enable the required services for your project from the Firebase dashboard.
Step 3: Add Firebase SDK to Your WordPress Site
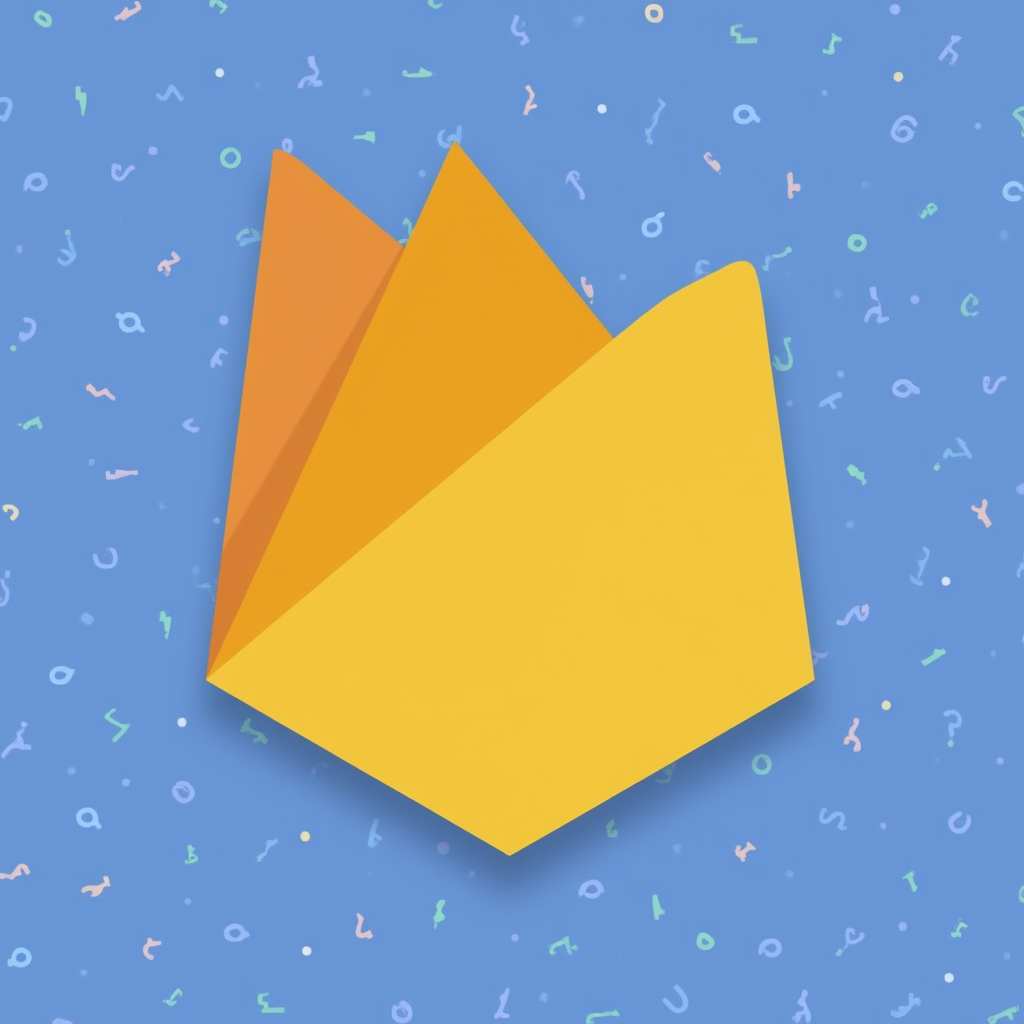
- Download the Firebase JavaScript SDK from the Firebase Console.
- Install the SDK in your WordPress theme or plugin:
- Navigate to the WordPress admin panel.
- Go to Appearance > Theme Editor or upload a custom plugin.
- Add the Firebase SDK scripts to your site’s header or footer file.
<script src="https://www.gstatic.com/firebasejs/9.0.0/firebase-app.js"></script>
<script src="https://www.gstatic.com/firebasejs/9.0.0/firebase-auth.js"></script>
<script src="https://www.gstatic.com/firebasejs/9.0.0/firebase-firestore.js"></script>
- Initialize Firebase in your code by including your project’s Firebase configuration:
const firebaseConfig = {
apiKey: "YOUR_API_KEY",
authDomain: "YOUR_PROJECT_ID.firebaseapp.com",
projectId: "YOUR_PROJECT_ID",
storageBucket: "YOUR_PROJECT_ID.appspot.com",
messagingSenderId: "YOUR_MESSAGING_SENDER_ID",
appId: "YOUR_APP_ID"
};
// Initialize Firebase
firebase.initializeApp(firebaseConfig);
Step 4: Set Up Authentication
Firebase Authentication can handle user sign-ins and logins effortlessly.
- Enable authentication methods:
- Navigate to Authentication > Sign-in Method in the Firebase Console.
- Choose the methods you want to enable (e.g., Email/Password, Google).
- Add a custom login form in WordPress or use a Firebase plugin to integrate authentication.
- Use the Firebase Authentication API to handle user authentication in WordPress:
firebase.auth().createUserWithEmailAndPassword(email, password)
.then((userCredential) => {
console.log('User signed up:', userCredential.user);
})
.catch((error) => {
console.error('Error signing up:', error);
});
Step 5: Integrate Firestore Database
Firestore is a cloud-based NoSQL database that provides real-time synchronization.
- Set up Firestore:
- Navigate to Firestore Database in the Firebase Console.
- Click Create Database and choose the appropriate mode (test or production).
- Use Firestore to save or retrieve WordPress data dynamically:
// Add a document to Firestore
firebase.firestore().collection('posts').add({
title: 'Hello Firebase',
content: 'This is a WordPress integration example.'
});
// Retrieve documents from Firestore
firebase.firestore().collection('posts').get()
.then((querySnapshot) => {
querySnapshot.forEach((doc) => {
console.log(doc.id, " => ", doc.data());
});
});
Step 6: Use Firebase Analytics
Track user behavior on your WordPress site with Firebase Analytics.
- Enable Analytics in your Firebase project.
- Add the Analytics SDK to your WordPress site:
<script src="https://www.gstatic.com/firebasejs/9.0.0/firebase-analytics.js"></script>
- Log events to track user interactions:
const analytics = firebase.analytics();
analytics.logEvent('page_view', { page_title: document.title });
Step 7: Test the Integration
After implementing the integration, thoroughly test all functionalities:
- User authentication and login/logout flows.
- Real-time database updates.
- Analytics event tracking.
- Compatibility with WordPress plugins and themes.
Optional: Use Plugins for Simplified Integration
For those who prefer a plugin-based approach, there are WordPress plugins like WP Firebase Authentication or Custom Firebase Integrations that can simplify the process.
Conclusion
Firebase wordpress integration unlocks a world of possibilities, from real-time updates to robust user authentication and analytics. By following this guide, you can leverage the best of both platforms to build a modern, dynamic website. With a bit of customization, your WordPress site can take full advantage of Firebase’s powerful backend services.